最近項(xiàng)目中,ui設(shè)計(jì)了個(gè)水波效果的背景動(dòng)畫,然而并沒有g(shù)if或svg動(dòng)畫,開始試著用css實(shí)現(xiàn)了一下,動(dòng)畫效果并不是很好,網(wǎng)上查了下基本都是用貝賽爾曲線實(shí)現(xiàn),想起以看的各種前波形圖,于是想著用三角函數(shù)圖像初略模擬一下
一、繪制sin函數(shù)圖像
sin函數(shù)表達(dá)式如下,
y=Asin(wx+φ)+h
其中 A表示振幅,ω表示角頻率(ω=2π/T,T為函數(shù)的周期),φ表示初相,h表示圖像向y軸正方向平移的長度 ;(這里需要注意一下:h在數(shù)學(xué)學(xué)的本來是表示向上平移的,但在canvas中采用的是屏幕坐標(biāo)系,即左上角為原點(diǎn),h則表示向下平移);
繪制代碼如下:
(1)添加canvas標(biāo)簽
<canvas id="canvas"></canvas>
(2)添加css樣式,設(shè)置canvas寬高
html,
body {
padding: 0;
margin: 0;
width: 100%;
height: 100%;
}
canvas {
width: 100%;
height: 100%;
}
(3)繪制函數(shù)圖像
var canvas = document.getElementById("canvas"),
ctx = canvas.getContext('2d'),
width = canvas.width = canvas.offsetWidth,
height = canvas.height = canvas.offsetHeight;
//聲明參數(shù)
var A=50,
W=1 / 50,
Q=0,
H= height / 2;
//繪圖方法
(function draw(){
ctx.clearRect(0, 0, width, height);//清空畫布
ctx.beginPath(); //開始路徑
ctx.strokeStyle="#000"; //設(shè)置線條顏色
ctx.lineWidth = 1; //設(shè)置線條寬度
ctx.moveTo(0, height /2); //起始點(diǎn)位置
for (let x = 0; x <= width; x++) {// 繪制x對(duì)應(yīng)y的
var y = A*Math.sin(W*x+Q) +H
ctx.lineTo(x, y)
}
ctx.stroke(); //繪制路徑
ctx.closePath(); //閉合路徑
})()
這樣我們可以得到以下圖像:
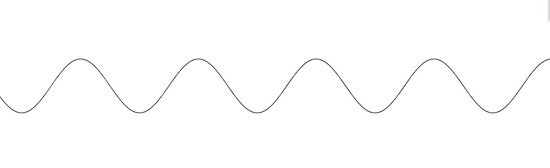
二、為函數(shù)圖像添加動(dòng)畫
上面得到的是是一個(gè)靜態(tài)的函數(shù)圖像,而我們一般見到的的波形圖或水波都是隨時(shí)間連續(xù)變化的,這里就要用到上一步中的參數(shù)相位φ,(js即代碼中的Q) ,我們將φ隨時(shí)間不斷增加或減小,即可得到不同時(shí)間的不同圖像;使用window.requestAnimationFrame實(shí)現(xiàn)幀動(dòng)畫;
修改以上代碼為:
var canvas = document.getElementById("canvas"),
ctx = canvas.getContext('2d'),
width = canvas.width = canvas.offsetWidth,
height = canvas.height = canvas.offsetHeight;
//聲明參數(shù)
var A=50,
W=1 / 50,
Q=0,
H= height / 2;
//繪圖方法
(function draw(){
ctx.clearRect(0, 0, width, height);//清空畫布
ctx.beginPath(); //開始路徑
ctx.strokeStyle="#000"; //設(shè)置線條顏色
ctx.lineWidth = 1; //設(shè)置線條寬度
ctx.moveTo(0, height /2); //起始點(diǎn)位置
for (let x = 0; x <= width; x++) {// 繪制x對(duì)應(yīng)y的
var y = A*Math.sin(W*x+Q) +H
ctx.lineTo(x, y)
}
ctx.stroke(); //繪制路徑
ctx.closePath(); //閉合路徑
})()
效果如下(渣渣截圖軟件):

三、繪制完整填充路徑
以上路徑雖有閉合,但卻不滿足我們需要填充的部分,直接填充效果如下:
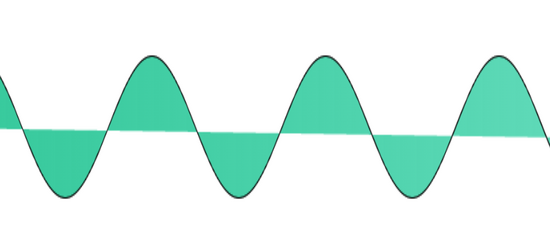
完整填充路徑應(yīng)如圖所示:
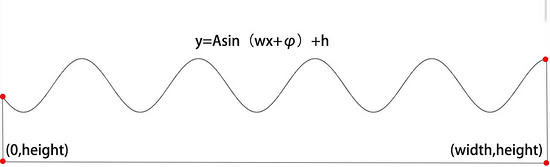
閉合路徑后創(chuàng)建一個(gè)漸變顏色,作為填充顏色,代碼如下:
var lingrad = ctx.createLinearGradient(0,0,width,0);
lingrad.addColorStop(0, 'rgba(0,186,128,0.8)');
lingrad.addColorStop(1, 'rgba(111,224,195,1)');
(function draw(){
window.requestAnimationFrame(draw);
ctx.clearRect(0, 0, width, height);
ctx.beginPath();
ctx.strokeStyle="#000";
ctx.fillStyle = lingrad;
ctx.lineWidth = 1;
ctx.moveTo(0, height /2);
Q+=speed;
for (let x = 0; x <= width; x++) {
var y = A*Math.sin(W*x+Q) +H;
ctx.lineTo(x, y);
}
ctx.lineTo(width, height);
ctx.lineTo(0, height);
ctx.fill();
ctx.closePath();
})()
效果如下:
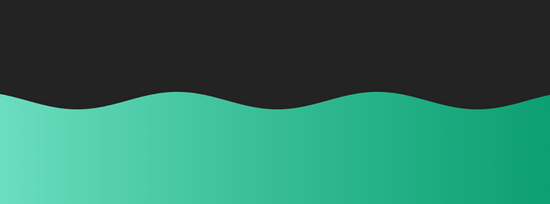
四、完善水波動(dòng)畫
1、首先可以對(duì)上面波形疊加一個(gè)頻率更高的波形,使波形無規(guī)矩
var s = 0.1*Math.sin(x/150)+1;
var y = A*Math.sin(W*x+Q) +H;
y=y*s;
2、再添加一個(gè)相位變化不同的波形,其漸變填充與上一個(gè)漸變方向相反使其形成相互重疊的陰影效果;并設(shè)置路徑重疊效果globalCompositeOperation;
var canvas = document.getElementById("canvas"),
ctx = canvas.getContext('2d'),
width = canvas.width = canvas.offsetWidth,
height = canvas.height = canvas.offsetHeight;
var A=30,
W=1 /200,
Q=0,
H= height / 2;
var A2=30,
W2=1/300,
Q2=0,
H2= height / 2;
var speed=-0.01;
var speed2=-0.02;
var lingrad = ctx.createLinearGradient(0,0,width,0);
lingrad.addColorStop(0, 'rgba(0,186,128,0.8)');
lingrad.addColorStop(1, 'rgba(111,224,195,1)');
var lingrad2 = ctx.createLinearGradient(0,0,width,0);
lingrad2.addColorStop(0,'rgba(111,224,195,1)');
lingrad2.addColorStop(1, 'rgba(0,186,128,0.8)');
(function draw(){
window.requestAnimationFrame(draw);
ctx.clearRect(0, 0, width, height);
ctx.beginPath();
ctx.fillStyle = lingrad;
ctx.moveTo(0, height /2);
//繪制第一個(gè)波形
Q+=speed;
for (let x = 0; x <= width; x++) {
var s = 0.1*Math.sin(x/150)+1;
var y = A*Math.sin(W*x+Q) +H;
y=y*s;
ctx.lineTo(x, y);
}
ctx.lineTo(width, height);
ctx.lineTo(0, height);
ctx.fill();
ctx.closePath()
//設(shè)置重疊效果
ctx.globalCompositeOperation = "destination-over"
//繪制第二個(gè)波形
ctx.beginPath();
ctx.fillStyle = lingrad2;
Q2+=speed2;
for (let x = 0; x < width; x++) {
var y = A2*Math.sin(x*W2+Q2) +H2;
ctx.lineTo(x, y);
}
ctx.lineTo(width,height);
ctx.lineTo(0,height);
ctx.fill()
ctx.closePath();
})()
以上就是本文的全部內(nèi)容,希望對(duì)大家的學(xué)習(xí)有所幫助,也希望大家多多支持腳本之家。