前言
最近在聽極客時(shí)間的課程,里面的講師極力推崇 pytest 框架,鄙視 unittest 框架,哈哈!然后查了些資料,發(fā)現(xiàn)了一條 python 鄙視鏈:pytest 鄙視 > unittest 鄙視 >robotframework 。
pytest 是 python 的第三方單元測(cè)試框架,比自帶 unittest 更簡(jiǎn)潔和高效,支持315種以上的插件,同時(shí)兼容 unittest 框架。這就使得我們?cè)?unittest 框架遷移到 pytest 框架的時(shí)候不需要重寫代碼。接下來(lái)我們?cè)谖闹衼?lái)對(duì)分析下 pytest 有哪些簡(jiǎn)潔、高效的用法。
一、安裝
首先使用 pip 安裝 pytest
查看 pytest 是否安裝成功
二、簡(jiǎn)單使用
1.創(chuàng)建 test_sample.py 文件,代碼如下:
#!/usr/bin/env python
# coding=utf-8
import pytest
def inc(x):
return x + 1
def test_answer():
assert inc(3) == 5
if __name__ =="__main__":
pytest.main()
執(zhí)行結(jié)果:
test_sample.py F [100%]
================================== FAILURES ===================================
_________________________________ test_answer _________________________________
def test_answer():
> assert inc(3) == 5
E assert 4 == 5
E + where 4 = inc(3)
test_sample.py:19: AssertionError
============================== 1 failed in 0.41s ==============================
從上面的例子可以看出,pytest 中斷言的用法直接使用 assert ,和 unittest 中斷言 self.assert 用法有所區(qū)別。
2.總結(jié)一下:使用 pytest 執(zhí)行測(cè)試需要遵行的規(guī)則:
- .py 測(cè)試文件必須以test_開頭(或者以_test結(jié)尾)
- 測(cè)試類必須以Test開頭,并且不能有 init 方法
- 測(cè)試方法必須以test_開頭
- 斷言必須使用 assert
三、fixture
pytest 提供的 fixture 實(shí)現(xiàn) unittest 中 setup/teardown 功能,可以在每次執(zhí)行case之前初始化數(shù)據(jù)。不同點(diǎn)是,fixture 可以只在執(zhí)行某幾個(gè)特定 case 前運(yùn)行,只需要在運(yùn)行 case 前調(diào)用即可。比 setup/teardown 使用起來(lái)更靈活。
1.fixture scope 作用范圍
先看下 fixture 函數(shù)的定義:
def fixture(scope="function", params=None, autouse=False, ids=None, name=None):
"""
:arg scope: 可選四組參數(shù):function(默認(rèn))、calss、module、package/session
:arg params: 一個(gè)可選的參數(shù)列表,它將導(dǎo)致多個(gè)參數(shù)調(diào)用fixture函數(shù)和所有測(cè)試使用它。
:arg autouse: 如果為True,則fixture func將為所有測(cè)試激活可以看到它。如果為False(默認(rèn)值),則需要顯式激活fixture。
:arg ids: 每個(gè)參數(shù)對(duì)應(yīng)的字符串id列表,因此它們是測(cè)試id的一部分。如果沒有提供id,它們將從參數(shù)中自動(dòng)生成。
:arg name: fixture的名稱。 這默認(rèn)為裝飾函數(shù)的名稱。 如果fixture在定義它的同一模塊中使用,夾具的功能名稱將被請(qǐng)求夾具的功能arg遮蔽; 解決這個(gè)問(wèn)題的一種方法是將裝飾函數(shù)命名 “fixture_ fixturename>”然后使用”@ pytest.fixture(name ='fixturename>')”。
"""
重點(diǎn)說(shuō)下 scope 四組參數(shù)的意義:
- function:每個(gè)方法(函數(shù))都會(huì)執(zhí)行一次。
- class:每個(gè)類都會(huì)執(zhí)行一次。類中有多個(gè)方法調(diào)用,只在第一個(gè)方法調(diào)用時(shí)執(zhí)行。
- module:一個(gè) .py 文件執(zhí)行一次。一個(gè).py 文件可能包含多個(gè)類和方法。
- package/session:多個(gè)文件調(diào)用一次,可以跨 .py 文件。
在所需要調(diào)用的函數(shù)前面加個(gè)裝飾器 @pytest.fixture()。舉一個(gè)簡(jiǎn)單的例子:
#!/usr/bin/env python
# coding=utf-8
import pytest
@pytest.fixture(scope='function')
def login():
print("登錄")
def test_1():
print('測(cè)試用例1')
def test_2(login):
print('測(cè)試用例2')
if __name__ =="__main__":
pytest.main(['test_sample.py','-s'])
執(zhí)行結(jié)果:
test_sample.py
測(cè)試用例1
.
登錄
測(cè)試用例2
.
============================== 2 passed in 0.07s ==============================
2.yield
我們剛剛實(shí)現(xiàn)了在每個(gè)用例之前執(zhí)行初始化操作,那么用例執(zhí)行完之后如需要 清除數(shù)據(jù)(或還原)操作,可以使用 yield 來(lái)實(shí)現(xiàn)。
#!/usr/bin/env python
# coding=utf-8
import pytest
@pytest.fixture(scope='function')
def login():
print("登錄")
yield
print("注銷登錄")
def test_1():
print('測(cè)試用例1')
def test_2(login):
print('測(cè)試用例2')
if __name__ =="__main__":
pytest.main(['test_sample.py','-s'])
執(zhí)行結(jié)果:
test_sample.py
測(cè)試用例1
.
登錄
測(cè)試用例2
.注銷登錄
============================== 2 passed in 0.08s ==============================
3.conftest
上面的案例都是寫在同一個(gè).py 文件內(nèi)的。倘若有多個(gè).py 文件需要調(diào)用 login() 方法,就必須把 login() 方法寫在外面,這里引用了conftest.py 配置文件。test_xxx.py 測(cè)試文件中無(wú)需 import conftest,pytest 會(huì)自動(dòng)搜索同級(jí)目錄中的 conftest.py 文件。
conftest.py 與 測(cè)試文件 目錄層級(jí)關(guān)系
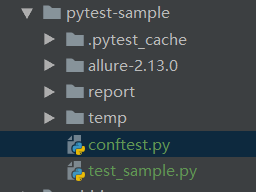
# 新建conftest.py,和 test_sample.py 同級(jí)目錄
import pytest
@pytest.fixture(scope='function')
def login():
print("登錄")
# test_sample.py 代碼如下
import pytest
def test_1():
print('測(cè)試用例1')
def test_2(login):
print('測(cè)試用例2')
if __name__ =="__main__":
pytest.main(['test_sample.py','-s'])
執(zhí)行結(jié)果:
test_sample.py
測(cè)試用例1
.
登錄
測(cè)試用例2
.
============================== 2 passed in 0.01s ==============================
四、重試機(jī)制
有的時(shí)候用例執(zhí)行失敗了,然后排查發(fā)現(xiàn)不是代碼問(wèn)題,可能和環(huán)境或者網(wǎng)絡(luò)不穩(wěn)定有關(guān)系,這個(gè)時(shí)候可以引入重試機(jī)制,排除一些外在因素。
1、安裝pytest-rerunfailures
pip3showpytest-rerunfailures
2、重試的兩種方法
1)使用裝飾器@pytest.mark.flaky(reruns=5,reruns_delay=2)
reruns :最大重試次數(shù)
reruns_delay :重試間隔時(shí)間,單位是秒
#!/usr/bin/env python
# coding=utf-8
import pytest
@pytest.mark.flaky(reruns=5, reruns_delay=2)
def test():
assert 0==1
if __name__ =="__main__":
pytest.main(['test_sample.py','-s'])
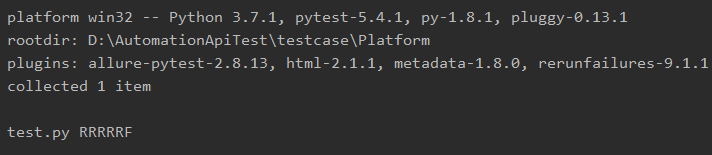
R表示用例失敗后正在重試,嘗試5次。
2)也可以使用命令行pytest--reruns5--reruns-delay 2 -s ,參數(shù)與裝飾器 @pytest.mark.flaky 一致,這個(gè)就不多說(shuō)了。
到此這篇關(guān)于Pytest 使用簡(jiǎn)介的文章就介紹到這了,更多相關(guān)Pytest 使用內(nèi)容請(qǐng)搜索腳本之家以前的文章或繼續(xù)瀏覽下面的相關(guān)文章希望大家以后多多支持腳本之家!
您可能感興趣的文章:- Python 測(cè)試框架unittest和pytest的優(yōu)劣
- 詳解如何使用Pytest進(jìn)行自動(dòng)化測(cè)試
- 詳解Pytest測(cè)試用例的執(zhí)行方法
- 自動(dòng)化測(cè)試Pytest單元測(cè)試框架的基本介紹
- pytest基本用法簡(jiǎn)介
- Python自動(dòng)化測(cè)試框架pytest的詳解安裝與運(yùn)行