一、錯誤案例
package main
import (
"fmt"
"time"
)
var TestMap map[string]string
func init() {
TestMap = make(map[string]string, 1)
}
func main() {
for i := 0; i 1000; i++ {
go Write("aaa")
go Read("aaa")
go Write("bbb")
go Read("bbb")
}
time.Sleep(5 * time.Second)
}
func Read(key string) {
fmt.Println(TestMap[key])
}
func Write(key string) {
TestMap[key] = key
}
上面代碼執(zhí)行大概率出現(xiàn)報錯:fatal error: concurrent map writes
二、問題分析
網(wǎng)上關(guān)于 golang 編程中 map 并發(fā)讀寫相關(guān)的資料很多,但總是都說成 并發(fā)讀寫 造成上面的錯誤,到底是 并發(fā)讀 還是 并發(fā)寫 造成的,這個很多資料都沒有說明。
我們把上面的案例分別在循環(huán)中注釋 Read 和 Write 函數(shù)的調(diào)用,分別測試 并發(fā)讀 和 并發(fā)寫;
循環(huán)次數(shù)分別測試了 100、1 w、100 w 次,并發(fā)讀操作絕對不會報上面的錯,而并發(fā)寫基本都會報錯。
因此,這個錯誤主要原因是:map 并發(fā)寫。
三、問題原因
為什么 map 并發(fā)寫會導致這個錯誤? 網(wǎng)絡(luò)上的相關(guān)文章也大都有說明。
因為 map 變量為 指針類型變量,并發(fā)寫時,多個協(xié)程同時操作一個內(nèi)存,類似于多線程操作同一個資源會發(fā)生競爭關(guān)系,共享資源會遭到破壞,因此golang 出于安全的考慮,拋出致命錯誤:fatal error: concurrent map writes。
四、解決方案
網(wǎng)上各路資料解決方案較多,主要思路是通過加鎖保證每個協(xié)程同步操作內(nèi)存。
github 上找到一個 concurrentMap 包,案例代碼修改如下:
package main
import (
"fmt"
cmap "github.com/orcaman/concurrent-map"
"time"
)
var TestMap cmap.ConcurrentMap
func init() {
TestMap = cmap.New()
}
func main() {
for i := 0; i 100; i++ {
go Write("aaa", "111")
go Read("aaa")
go Write("bbb", "222")
go Read("bbb")
}
time.Sleep(5 * time.Second)
}
func Read(key string) {
if v, ok := TestMap.Get(key); ok {
fmt.Printf("鍵值為 %s 的值為:%s", key, v)
} else {
fmt.Printf("鍵值不存在")
}
}
func Write(key string, value string) {
TestMap.Set(key, value)
}
五、思考總結(jié)
因為我是以 PHP 打開的編程世界,PHP 語言只有單線程,且不涉及指針操作,變量類型也是弱變量,以 PHP 編程思維剛開始接觸 Golang 時還比較容易上手,但越往后,語言的特性區(qū)別就體現(xiàn)得越來越明顯,思維轉(zhuǎn)變就越來越大,對我來說是打開了一個新世界。
像本文出現(xiàn)的錯誤案例,也是因為自己沒有多線程編程的思維基礎(chǔ),導致對這種問題不敏感,還是花了蠻多時間理解的。希望對和我有相似學習路線的朋友提供到一些幫助。
補充:Golang Map并發(fā)處理機制(sync.Map)
Go語言中的Map在并發(fā)情況下,只讀是線程安全的,同時讀寫線程不安全。
示例:
package main
import (
"fmt"
)
var m = make(map[int]int)
func main() {
//寫入操作
i:=0
go func() {
for{
i++
m[1]=i
}
}()
//讀操作
go func() {
for{
fmt.Println(m[1])
}
}()
//無限循環(huán),讓并發(fā)程序在后臺運行
for {
;
}
}
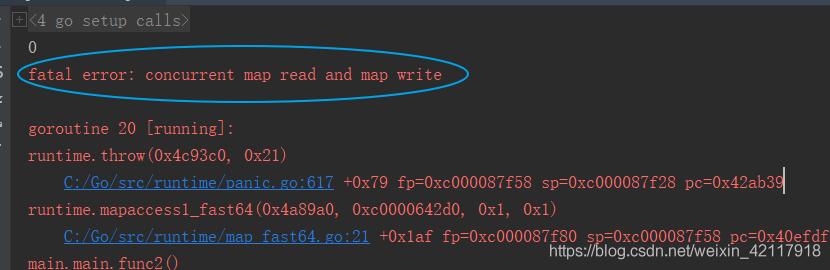
從以上示例可以看出,不斷地對map進行讀和寫,會出現(xiàn)錯誤。主要原因是對map進行讀和寫發(fā)生了競態(tài)問題。map內(nèi)部會對這種并發(fā)操作進行檢查并提前發(fā)現(xiàn)。
如果確實需要對map進行并發(fā)讀寫操作,可以采用加鎖機制、channel同步機制,但這樣性能并不高。
Go語言在1.9版本中提供了一種效率較高的并發(fā)安全的sync.Map。
sync.Map結(jié)構(gòu)如下:
The zero Map is empty and ready for use. A Map must not be copied after first use.
type Map struct {
mu Mutex
misses int
}
// Load returns the value stored in the map for a key, or nil if no
// value is present.
// The ok result indicates whether value was found in the map.
func (m *Map) Load(key interface{}) (value interface{}, ok bool) {
}
// Store sets the value for a key.
func (m *Map) Store(key, value interface{}) {
}
// LoadOrStore returns the existing value for the key if present.
// Otherwise, it stores and returns the given value.
// The loaded result is true if the value was loaded, false if stored.
func (m *Map) LoadOrStore(key, value interface{}) (actual interface{}, loaded bool) {
}
// Delete deletes the value for a key.
func (m *Map) Delete(key interface{}) {
}
// Range calls f sequentially for each key and value present in the map.
// If f returns false, range stops the iteration.
//
// Range does not necessarily correspond to any consistent snapshot of the Map's
// contents: no key will be visited more than once, but if the value for any key
// is stored or deleted concurrently, Range may reflect any mapping for that key
// from any point during the Range call.
//
// Range may be O(N) with the number of elements in the map even if f returns
// false after a constant number of calls.
func (m *Map) Range(f func(key, value interface{}) bool) {
}
func (m *Map) missLocked() {
}
func (m *Map) dirtyLocked() {
}
其實,sync.Map內(nèi)部還是進行了加鎖機制,不過進行了一定的優(yōu)化。
sync.Map使用示例:
package main
import (
"fmt"
"sync"
"time"
)
var m1 sync.Map
func main() {
i := 0
go func() {
for {
i++
m1.Store(1, i)
time.Sleep(1000)
}
}()
go func() {
for{
time.Sleep(1000)
fmt.Println(m1.Load(1))
}
}()
for {
;
}
}
成功運行效果如下:
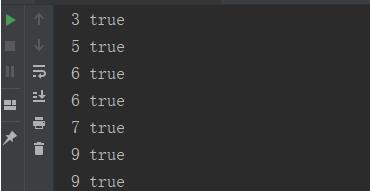
以上為個人經(jīng)驗,希望能給大家一個參考,也希望大家多多支持腳本之家。如有錯誤或未考慮完全的地方,望不吝賜教。
您可能感興趣的文章:- 關(guān)于golang高并發(fā)的實現(xiàn)與注意事項說明
- 基于Golang 高并發(fā)問題的解決方案
- 使用golang編寫一個并發(fā)工作隊列
- golang 并發(fā)編程之生產(chǎn)者消費者詳解
- Golang 并發(fā)以及通道的使用方式
- 淺談golang并發(fā)操作變量安全的問題
- golang中的并發(fā)和并行