目的
Facade通過嵌入多個(當然,有時只有一個)接口來解耦訪客與子系統(tǒng),同時也為了降低復雜度。
- Facade 不會禁止你訪問子系統(tǒng)
- 你可以(應該)為一個子系統(tǒng)提供多個 Facade
因此一個好的 Facade 里面不會有 new 。如果每個方法里都要構造多個對象,那么它就不是 Facade,而是生成器或者[抽象|靜態(tài)|簡單] 工廠 [方法]。
優(yōu)秀的 Facade 不會有 new,并且構造函數(shù)參數(shù)是接口類型的。如果你需要創(chuàng)建一個新實例,則在參數(shù)中傳入一個工廠對象。
UML
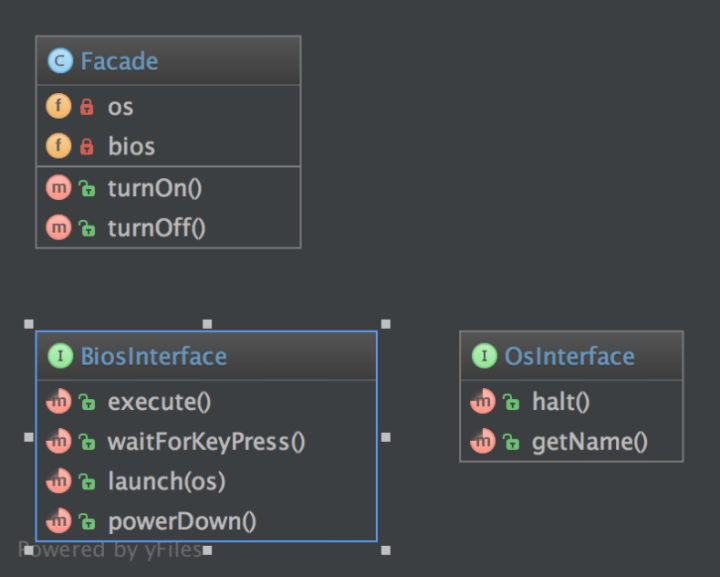
代碼
Facade.php
?php
namespace DesignPatterns\Structural\Facade;
class Facade
{
/**
* @var OsInterface
* 定義操作系統(tǒng)接口變量。
*/
private $os;
/**
* @var BiosInterface
* 定義基礎輸入輸出系統(tǒng)接口變量。
*/
private $bios;
/**
* @param BiosInterface $bios
* @param OsInterface $os
* 傳入基礎輸入輸出系統(tǒng)接口對象 $bios 。
* 傳入操作系統(tǒng)接口對象 $os 。
*/
public function __construct(BiosInterface $bios, OsInterface $os)
{
$this->bios = $bios;
$this->os = $os;
}
/**
* 構建基礎輸入輸出系統(tǒng)執(zhí)行啟動方法。
*/
public function turnOn()
{
$this->bios->execute();
$this->bios->waitForKeyPress();
$this->bios->launch($this->os);
}
/**
* 構建系統(tǒng)關閉方法。
*/
public function turnOff()
{
$this->os->halt();
$this->bios->powerDown();
}
}
OsInterface.php
?php
namespace DesignPatterns\Structural\Facade;
/**
* 創(chuàng)建操作系統(tǒng)接口類 OsInterface 。
*/
interface OsInterface
{
/**
* 聲明關機方法。
*/
public function halt();
/**
* 聲明獲取名稱方法,返回字符串格式數(shù)據(jù)。
*/
public function getName(): string;
}
BiosInterface.php
?php
namespace DesignPatterns\Structural\Facade;
/**
* 創(chuàng)建基礎輸入輸出系統(tǒng)接口類 BiosInterface 。
*/
interface BiosInterface
{
/**
* 聲明執(zhí)行方法。
*/
public function execute();
/**
* 聲明等待密碼輸入方法
*/
public function waitForKeyPress();
/**
* 聲明登錄方法。
*/
public function launch(OsInterface $os);
/**
* 聲明關機方法。
*/
public function powerDown();
}
測試
Tests/FacadeTest.php
?php
namespace DesignPatterns\Structural\Facade\Tests;
use DesignPatterns\Structural\Facade\Facade;
use DesignPatterns\Structural\Facade\OsInterface;
use PHPUnit\Framework\TestCase;
/**
* 創(chuàng)建自動化測試單元 FacadeTest 。
*/
class FacadeTest extends TestCase
{
public function testComputerOn()
{
/** @var OsInterface|\PHPUnit_Framework_MockObject_MockObject $os */
$os = $this->createMock('DesignPatterns\Structural\Facade\OsInterface');
$os->method('getName')
->will($this->returnValue('Linux'));
$bios = $this->getMockBuilder('DesignPatterns\Structural\Facade\BiosInterface')
->setMethods(['launch', 'execute', 'waitForKeyPress'])
->disableAutoload()
->getMock();
$bios->expects($this->once())
->method('launch')
->with($os);
$facade = new Facade($bios, $os);
// 門面接口很簡單。
$facade->turnOn();
// 但你也可以訪問底層組件。
$this->assertEquals('Linux', $os->getName());
}
}
以上就是淺談PHP設計模式之門面模式Facade的詳細內容,更多關于PHP設計模式之門面模式Facade的資料請關注腳本之家其它相關文章!
您可能感興趣的文章:- PHP設計模式(觀察者模式)
- 淺談PHP設計模式之對象池模式Pool
- 詳解PHP設計模式之依賴注入模式
- PHP設計模式之迭代器模式的使用
- 詳解PHP八大設計模式
- PHP設計模式之原型模式示例詳解
- PHP設計模式之命令模式示例詳解
- PHP八大設計模式案例詳解