文件上傳和下載功能是Java Web必備技能,很實(shí)用。
本文使用的是Apache下的著名的文件上傳組件
org.apache.commons.fileupload 實(shí)現(xiàn)
下面結(jié)合最近看到的一些資料以及自己的嘗試,先寫第一篇文件上傳。后續(xù)會(huì)逐步實(shí)現(xiàn)下載,展示文件列表,上傳信息持久化等。
廢話少說,直接上代碼
第一步、引用jar包,設(shè)置上傳目錄
commons-fileupload-1.3.1.jar
commons-io-2.4.jar
上傳目錄:WEB-INF/tempFiles和WEB-INF/uploadFiles
第二步、編寫JSP頁(yè)面
%@ page contentType="text/html;charset=UTF-8" language="java" %>
%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
html>
head>
title>文件上傳測(cè)試/title>
/head>
body>
form method="POST" enctype="multipart/form-data" action="%=request.getContextPath()%>/UploadServlet">
文件: input type="file" name="upfile">br/>
br/>
input type="submit" value="上傳">
/form>
c:if test="${not empty errorMessage}">
input type="text" id="errorMessage" value="${errorMessage}" style="color:red;" disabled="disabled">
/c:if>
/body>
/html>
第三步、編寫Servlet,處理文件上傳的核心
package servlet;
import org.apache.commons.fileupload.FileItem;
import org.apache.commons.fileupload.FileUploadBase;
import org.apache.commons.fileupload.FileUploadException;
import org.apache.commons.fileupload.ProgressListener;
import org.apache.commons.fileupload.disk.DiskFileItemFactory;
import org.apache.commons.fileupload.servlet.ServletFileUpload;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.util.Calendar;
import java.util.Iterator;
import java.util.List;
import java.util.UUID;
/**
* 處理文件上傳
*
* @author xusucheng
* @create 2017-12-27
**/
@WebServlet("/UploadServlet")
public class UploadServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
//設(shè)置文件上傳基本路徑
String savePath = this.getServletContext().getRealPath("/WEB-INF/uploadFiles");
//設(shè)置臨時(shí)文件路徑
String tempPath = this.getServletContext().getRealPath("/WEB-INF/tempFiles");
File tempFile = new File(tempPath);
if (!tempFile.exists()) {
tempFile.mkdir();
}
//定義異常消息
String errorMessage = "";
//創(chuàng)建file items工廠
DiskFileItemFactory factory = new DiskFileItemFactory();
//設(shè)置緩沖區(qū)大小
factory.setSizeThreshold(1024 * 100);
//設(shè)置臨時(shí)文件路徑
factory.setRepository(tempFile);
//創(chuàng)建文件上傳處理器
ServletFileUpload upload = new ServletFileUpload(factory);
//監(jiān)聽文件上傳進(jìn)度
ProgressListener progressListener = new ProgressListener() {
public void update(long pBytesRead, long pContentLength, int pItems) {
System.out.println("正在讀取文件: " + pItems);
if (pContentLength == -1) {
System.out.println("已讀?。?" + pBytesRead + " 剩余0");
} else {
System.out.println("文件總大?。? + pContentLength + " 已讀?。? + pBytesRead);
}
}
};
upload.setProgressListener(progressListener);
//解決上傳文件名的中文亂碼
upload.setHeaderEncoding("UTF-8");
//判斷提交上來的數(shù)據(jù)是否是上傳表單的數(shù)據(jù)
if (!ServletFileUpload.isMultipartContent(request)) {
//按照傳統(tǒng)方式獲取數(shù)據(jù)
return;
}
//設(shè)置上傳單個(gè)文件的大小的最大值,目前是設(shè)置為1024*1024字節(jié),也就是1MB
upload.setFileSizeMax(1024 * 1024);
//設(shè)置上傳文件總量的最大值,最大值=同時(shí)上傳的多個(gè)文件的大小的最大值的和,目前設(shè)置為10MB
upload.setSizeMax(1024 * 1024 * 10);
try {
//使用ServletFileUpload解析器解析上傳數(shù)據(jù),解析結(jié)果返回的是一個(gè)ListFileItem>集合,每一個(gè)FileItem對(duì)應(yīng)一個(gè)Form表單的輸入項(xiàng)
ListFileItem> items = upload.parseRequest(request);
IteratorFileItem> iterator = items.iterator();
while (iterator.hasNext()) {
FileItem item = iterator.next();
//判斷jsp提交過來的是不是文件
if (item.isFormField()) {
errorMessage = "請(qǐng)?zhí)峤晃募?;
break;
} else {
//文件名
String fileName = item.getName();
if (fileName == null || fileName.trim() == "") {
System.out.println("文件名為空!");
}
//處理不同瀏覽器提交的文件名帶路徑問題
fileName = fileName.substring(fileName.lastIndexOf("\\") + 1);
//文件擴(kuò)展名
String fileExtension = fileName.substring(fileName.lastIndexOf(".") + 1);
//判斷擴(kuò)展名是否合法
if (!validExtension(fileExtension)) {
errorMessage = "上傳文件非法!";
item.delete();
break;
}
//獲得文件輸入流
InputStream in = item.getInputStream();
//得到保存文件的名稱
String saveFileName = createFileName(fileName);
//得到文件保存路徑
String realFilePath = createRealFilePath(savePath, saveFileName);
//創(chuàng)建文件輸出流
FileOutputStream out = new FileOutputStream(realFilePath);
//創(chuàng)建緩沖區(qū)
byte buffer[] = new byte[1024];
int len = 0;
while ((len = in.read(buffer)) > 0) {
//寫文件
out.write(buffer, 0, len);
}
//關(guān)閉輸入流
in.close();
//關(guān)閉輸出流
out.close();
//刪除臨時(shí)文件 TODO
item.delete();
//將上傳文件信息保存到附件表中 TODO
}
}
} catch (FileUploadBase.FileSizeLimitExceededException e) {
e.printStackTrace();
request.setAttribute("errorMessage", "單個(gè)文件超出最大值!??!");
request.getRequestDispatcher("pages/upload/upload.jsp").forward(request, response);
return;
} catch (FileUploadBase.SizeLimitExceededException e) {
e.printStackTrace();
request.setAttribute("errorMessage", "上傳文件的總的大小超出限制的最大值?。?!");
request.getRequestDispatcher("pages/upload/upload.jsp").forward(request, response);
return;
} catch (FileUploadException e) {
e.printStackTrace();
request.setAttribute("errorMessage", "文件上傳失?。。?!");
request.getRequestDispatcher("pages/upload/upload.jsp").forward(request, response);
return;
}
request.setAttribute("errorMessage", errorMessage);
request.getRequestDispatcher("pages/upload/upload.jsp").forward(request, response);
}
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
private boolean validExtension(String fileExtension) {
String[] exts = {"jpg", "txt", "doc", "pdf"};
for (int i = 0; i exts.length; i++) {
if (fileExtension.equals(exts[i])) {
return true;
}
}
return false;
}
private String createFileName(String fileName) {
return UUID.randomUUID().toString() + "_" + fileName;
}
/**
* 根據(jù)基本路徑和文件名稱生成真實(shí)文件路徑,基本路徑\\年\\月\\fileName
*
* @param basePath
* @param fileName
* @return
*/
private String createRealFilePath(String basePath, String fileName) {
Calendar today = Calendar.getInstance();
String year = String.valueOf(today.get(Calendar.YEAR));
String month = String.valueOf(today.get(Calendar.MONTH) + 1);
String upPath = basePath + File.separator + year + File.separator + month + File.separator;
File uploadFolder = new File(upPath);
if (!uploadFolder.exists()) {
uploadFolder.mkdirs();
}
String realFilePath = upPath + fileName;
return realFilePath;
}
}
第四步、測(cè)試
http://localhost:8080/helloweb/pages/upload/upload.jsp
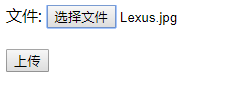

以上就是本文的全部?jī)?nèi)容,希望對(duì)大家的學(xué)習(xí)有所幫助,也希望大家多多支持腳本之家。
您可能感興趣的文章:- Jsp頁(yè)面實(shí)現(xiàn)文件上傳下載類代碼
- jsp中點(diǎn)擊圖片彈出文件上傳界面及預(yù)覽功能的實(shí)現(xiàn)
- jsp實(shí)現(xiàn)文件上傳下載的程序示例
- AJAX和JSP實(shí)現(xiàn)的基于WEB的文件上傳的進(jìn)度控制代碼
- jsp文件上傳與下載實(shí)例代碼
- jsp中點(diǎn)擊圖片彈出文件上傳界面及實(shí)現(xiàn)預(yù)覽實(shí)例詳解
- 利用jsp+Extjs實(shí)現(xiàn)動(dòng)態(tài)顯示文件上傳進(jìn)度
- jsp 文件上傳瀏覽,支持ie6,ie7,ie8
- servlet+JSP+mysql實(shí)現(xiàn)文件上傳的方法
- JSP實(shí)現(xiàn)文件上傳功能